PRINT AN INTEGER IN C
Print an integer in C
Print an integer in C language: a user will input an integer, and it will be printed. Input is done using scanf function and the number is printed on screen using printf.
C program to print an integer
- #include <stdio.h>
- int main()
- {
- int a;
- printf("Enter an integer\n");
- scanf("%d", &a);
- printf("The integer is %d\n", a);
- return 0;
- }
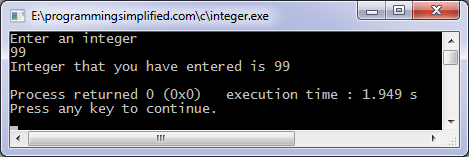
- #include <stdio.h>
- int main()
- {
- int c;
- for (c = 1; c <= 100; c++)
- printf("%d ", c);
- return 0;
- }
In C language we have data types for different types of data, for integers, it is int, for characters it is char, for floating point data it's float and so on. For large integers, you can use long or long long data type. To store integers which are larger than (2^18-1) which is the range of long long data type you may use strings. In the below program we store an integer in a string and then display it.
C program to store an integer in a string
- #include <stdio.h>
- int main ()
- {
- char n[1000];
- printf("Input an integer\n");
- scanf("%s", n);
- printf("%s", n);
- return 0;
- }
- Input an integer
- 12345678909876543210123456789
- 12345678909876543210123456789
Comments
Post a Comment